Go (Golang) Programming The Complete Go Bootcamp 2023
17:49:29 Inglés Free 27/05/2024 155 videos
Descripción del curso
Fully updated for 2021. This Go Programming course covers every major topic, including Pointers, Methods, and Interfaces (Go OOP), Concurrency In-Depth (Goroutines, Channels, Mutexes, WaitGroups), Go Packages and Modules, and many more! This course IS NOT like any other Go Programming course you can take online. At the end of this course, you will MASTER all the Golang key concepts starting from scratch and you'll be in the top Go Programmers.
This is a brand new Go Programming course just updated and is a perfect match for both beginners and experienced developers!
Welcome to this practical Go Programming course for learning Go, the language created to solve "Google-size" problems.
Go (Golang) will be one of the most in-demand programming languages across the job market in the near future! Go is on a Trajectory to Become the Next Enterprise Programming Language. Cutting-Edge Technologies and Software are already written in Go, for example, Docker, Kubernetes, Terraform, or Ethereum. In addition to Google, Golang is used by Uber, Netflix, Medium, Pinterest, Slack, SoundCloud, Dropbox and so many more!
Why this Go Programming course?
This Go (Golang) course is a unique experience on Udemy. There are many other Go courses you can choose from, but this course is completely different.
For every Go language key concept, you'll get NOT ONLY a video but also:
1. Tens of Quizzes
2. Practice Exercises and Challenges
3. Coding Section Full of Examples
4. Slides with main points
Curriculum
Section 1: Module 1
About the Instructor
udemy
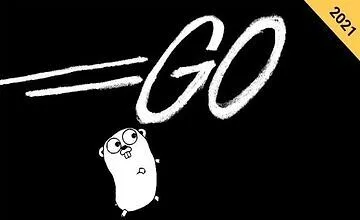
$0.00
$8.00 Que esta incluido?
- Streaming Multiplataforma
- Acceso de por vida
- Soporte al cliente
- Actualizaciones gratuitas