Master Spring 6 Spring Boot 3 REST JPA Hibernate
36:15:18 Inglés Free 01/11/2023 248 videos
Descripción del curso
'Master Spring framework, Spring Boot, REST, JPA, Hibernate' course will help in understanding about Spring framework and how to build web applications, Rest Services using Spring, Spring MVC, SpringBoot, Thymeleaf, Spring JDBC, Spring Data JPA etc. By the end of this course, students will understand all the below topics:
- What is Spring framework ?
- Spring Vs Java EE
- Evolution of Spring and release timeline of Spring
- Different projects inside Spring
- Spring Core Concepts like Inversion of Control (IoC), Dependency Injection (DI) & Aspect-Oriented Programming (AOP)
- Different approaches of Beans creation inside Spring framework
- Bean Scopes inside Spring framework
- Autowiring of the Spring Beans
- Introduction to MVC pattern & overview of web apps
- Spring MVC internal architecture & how to create web applications using Spring MVC & Thymeleaf
- Spring MVC Validations
- How to build dynamic web apps using Thymeleaf & Spring
- Thymeleaf integration with Spring, Spring MVC, Spring Security
- Deep dive on Spring Boot, Auto-configuration
- Spring Boot Dev Tools
- Spring Boot H2 Database
- Securing web applications using Spring Security
- Authentication , Authorization, Role based access
- Cross-Site Request Forgery (CSRF) & Cross-Origin Resource Sharing (CORS)
- Database create, read, update, delete operations using Spring JDBC
- Introduction to ORM frameworks & database create, read, update, delete operations using Spring Data JPA/Hibernate
- Derived Query methods in JPA
- OneToOne, OneToMany, ManyToOne, ManyToMany mappings inside JPA/Hibernate
- Sorting, Pagination, JPQL inside Spring Data JPA
- Building Rest Services inside Spring
- Consuming Rest Services using OpenFeign, Web Client, RestTemplate
- Spring Data Rest & HAL Explorer
- Logging inside Spring applications
- Properties Configuration inside Spring applications
- Profiles inside Spring Boot applications
- Conditional Bean creation using Profiles
- Monitoring Spring Boot applications using SpringBoot Actuator & Spring Boot Admin
Curriculum
Section 1: Module 1
- 02 - Introduction to the course & Agenda of the course 14:36
- 03 - Details of Source Code, PDF Content & other instructions for the course 10:10
- 04 - What is Spring ? 08:16
- 05 - Jakarta EE Vs Spring 12:06
- 06 - Introduction to Spring Core 02:23
- 07 - Introduction to Inversion of Control (IoC) & Dependency Injection (DI) 03:52
- 08 - Demo of Inversion of Control (IoC) & Dependency Injection (DI) 11:15
- 09 - Advantages of Inversion of Control & Dependency Injection 07:03
- 10 - Introductions to Beans, Context and SpEL 06:09
- 11 - Introduction to Spring IoC Container 06:42
- 12 - Installation of Maven 09:06
- 13 - Creating Maven Project 17:05
- 14 - Creating Beans using @Bean annotation 15:50
- 15 - Understanding NoUniqueBeanDefinitionException in Spring 08:09
- 16 - Providing a custom name to the bean 04:41
- 17 - Understanding @Primary Annotation inside Spring 05:57
- 18 - Creating Beans using @Component annotation 08:34
- 19 - Stereotype Annotations in Spring 05:56
- 20 - Comparison between @Bean Vs @Component 05:35
- 21 - Understanding @PostConstruct Annotation 06:05
- 22 - Understanding @PreDestroy Annotation 05:04
- 23 - Creating Beans programmatically using registerBean() 10:04
- 24 - Creating Beans using XML Configurations 07:33
- 25 - Why should we use frameworks 11:46
- 26 - Introduction to Spring Projects - Part 1 09:16
- 27 - Introduction to Spring Projects - Part 2 06:17
- 28 - Introduction to wiring & auto-wiring inside Spring 07:54
- 29 - Wiring Beans using method call 09:53
- 30 - Wiring Beans using method parameters 03:37
- 31 - Wiring Beans using @Autowired on class fields 09:06
- 32 - Wiring Beans using @Autowired on setter method 04:49
- 33 - Wiring Beans using @Autowired on constructor 05:43
- 34 - Deep dive of Autowiring inside Spring - Theory 07:34
- 35 - Deep dive of Autowiring inside Spring - Coding example 05:57
- 36 - Understanding and Avoiding Circular dependencies 04:55
- 37 - Problem Statement for Assignment related to Beans, Autowiring and DI 04:43
- 38 - Solution for Assignment related to Beans, Autowiring and DI 15:34
- 39 - Introduction to Bean Scopes inside Spring 03:24
- 40 - Deepdive on Singleton Bean scope 07:03
- 41 - What is a Race Condition 03:38
- 42 - Usecases of Singleton Bean scope 04:46
- 43 - Deepdive of Eager and Lazy instantiation of Singleton scope 05:57
- 44 - Demo of Eager and Lazy instantiation of Singleton bean 04:29
- 45 - Eager Initialization Vs Lazy Initialization 03:57
- 46 - Deepdive of Prototype Bean scope 05:43
- 47 - Singleton Beans Vs Prototype Beans 04:11
- 48 - Introduction to Aspect Oriented Programming (AOP) 05:45
- 49 - Understanding the problems inside web applications with out AOP 07:13
- 50 - Understanding & Running the Application with out AOP 07:13
- 51 - AOP Jargons 05:16
- 52 - Weaving inside AOP 03:14
- 53 - Type of Advices inside AOP 04:15
- 54 - Configuring Advices inside AOP - Theory 11:33
- 55 - Configuring @Around advice 10:56
- 56 - Configuring @Before advice 07:30
- 57 - Configuring @AfterThrowing and @AfterReturning advices 07:24
- 58 - Configuring Advices inside AOP with Annotations approach 03:15
- 59 - Demo of Configuring Advices inside AOP with Annotations approach 05:43
- 60 - Quick Introduction about Web Applications 07:38
- 61 - Role of Servlets inside Web Applications 06:46
- 62 - Evolution of Web Apps inside Java ecosystem 04:16
- 63 - Types of Web Apps we can build with Spring 06:35
- 64 - Introduction to Spring Boot - The Hero of Spring framework 10:42
- 65 - Spring Boot Important features 11:03
- 66 - Creating simple web application using Spring Boot 14:24
- 67 - Running simple web application using Spring Boot 13:57
- 68 - Changing the default server port & context path of SpringBoot Web application 05:31
- 69 - Random server port number inside SpringBoot 04:09
- 70 - Demo of SpringBoot Autoconfiguration 11:42
- 71 - Quick recap 05:08
- 72 - Quick Tip - Mapping multiple paths inside Spring Web Application 13:12
- 73 - Introduction to Thymeleaf 08:39
- 74 - Building dynamic content using Thymeleaf 09:59
- 75 - Disabling Thymeleaf template caching 07:04
- 76 - Introduction to Spring Boot DevTools 07:38
- 77 - Implemetation & Demo of Spring Boot DevTools 07:39
- 78 - Building Home Page of EazySchool Web Application 07:24
- 79 - Understanding the Home Page source code of EazySchool 05:56
- 80 - Deep Dive of Spring MVC Internal architecture 12:28
- 81 - Separation of Header and Footer code using Thymeleaf replace tag 08:15
- 82 - Building Courses Web Page of Eazy School Web Application 12:08
- 83 - Quick Tip - Resolving Build & Cache issues inside maven projects 02:13
- 84 - Building About Page of Eazy School Web Application 06:48
- 85 - Building Contact Page of Eazy School Web Application 05:26
- 86 - Submit information from Contact page using @RequestParam 10:54
- 87 - Submit information from Contact page using POJO object 08:31
- 88 - Define actions for all the links in the Home & Footer page 06:19
- 89 - Building Holidays Page of Eazy School Web Application 12:36
- 90 - Introduction to Lombok library 07:00
- 91 - Implementing Lombok inside Eazy School Web App 06:39
- 92 - Demo of @Slf4j annotation from Lombok library 03:57
- 93 - Accepting Query Params using @RequestParam annotation - Theory 08:47
- 94 - Accepting Query Params using @RequestParam annotation - Coding 12:05
- 95 - Accepting Path Params using @PathVariable annotation - Theory 05:23
- 96 - Accepting Path Params using @PathVariable annotation - Coding 07:19
- 97 - Importance of Validations inside Web Applications 12:00
- 98 - Introduction to Java Bean Validations 10:10
- 99 - Adding Bean Validation annotations inside Contact POJO class 10:14
- 100 - Adding Bean Validation related changes inside EazySchool Web Application 12:17
- 101 - Demo of Bean Validations inside Contact form Page 06:49
- 102 - Introduction to Spring Web Scopes 07:02
- 103 - Use Cases of Spring Web Scopes 09:27
- 104 - Demo of @RequestScope inside Eazy School Web Application 06:32
- 105 - Demo of @SessionScope inside Eazy School Web Application 06:29
- 106 - Demo of @ApplicationScope inside Eazy School Web Application 05:48
- 107 - Introduction to Spring Security 07:17
- 108 - Deepdive of Authentication Vs Authorization 05:48
- 109 - Demo of Spring Security inside Eazy School Web App with default behavior 06:28
- 110 - Configure custom credentials inside Spring Security 04:29
- 111 - Understanding default security configurations inside Spring Security framework 05:55
- 112 - Configure permitAll() inside Web App using Spring Security 07:15
- 113 - Configure denyAll() inside Web App using Spring Security 05:48
- 114 - Configure custom security configurations using Spring Security 10:58
- 115 - Demo of CSRF protection & CSRF Disable inside Spring Security framework 09:34
- 116 - Configure multiple users using inMemoryAuthentication() of Spring Security 09:27
- 117 - Implement Login & Logout inside Web App - Part 1 07:11
- 118 - Implement Login & Logout inside Web App - Part 2 09:51
- 119 - Implement Login & Logout inside Web App - Part 3 05:16
- 120 - Demo of integration between ThymeLeaf & Spring Security 10:37
- 121 - Introduction to @ControllerAdvice & @ExceptionHandler annotations 09:00
- 122 - Demo of @ControllerAdvice & @ExceptionHandler annotations 10:43
- 123 - Deep dive of CSRF attack 12:14
- 124 - Solution for CSRF attack - Theory 10:22
- 125 - Solution for CSRF attack - Coding 11:26
- 126 - Introduction to in-memory H2 Database of Spring Boot 08:38
- 127 - Setup H2 Database inside a Spring Boot web application 16:37
- 128 - Introduction to JDBC & problems with it 09:42
- 129 - Introduction to Spring JDBC 10:40
- 130 - Deep dive on usage of JdbcTemplate 08:01
- 131 - Saving Contact Message into DB using JdbcTemplate Insert operation 14:26
- 132 - Display Contact messages from DB using JdbcTemplate select operation - Part 1 06:52
- 133 - Display Contact messages from DB using JdbcTemplate select operation - Part 2 15:41
- 134 - Update Contact messages status using JdbcTemplate update operation 09:41
- 135 - Implementing AOP inside Eazy School Web Application 08:23
- 136 - Display list of Holidays from H2 Database using JdbcTemplate 16:29
- 137 - Setup MYSQL DB inside AWS - Part 1 08:28
- 138 - Setup MYSQL DB inside AWS - Part 2 09:41
- 139 - Migrate from H2 Database to MYSQL Database 09:09
- 140 - Demo of MYSQL Database changes inside Eazy School Web App 09:39
- 141 - Problems with Spring JDBC & how ORM frameworks solve these problems 06:08
- 142 - Introduction to Spring Data 06:46
- 143 - Deepdive on Repository,CrudRepository,PagingAndSortingRepository,JpaRepository 16:50
- 144 - Introduction to Spring Data JPA 12:26
- 145 - Migrate from Spring JDBC to Spring Data JPA - Part 1 11:07
- 146 - Migrate from Spring JDBC to Spring Data JPA - Part 2 13:12
- 147 - Migrate from Spring JDBC to Spring Data JPA - Part 3 08:28
- 148 - Migrate from Spring JDBC to Spring Data JPA - Part 4 09:50
- 149 - Deep dive on derived query methods inside Spring Data JPA 09:20
- 150 - Introduction of Auditing Support by Spring Data JPA 12:50
- 151 - Implement automatic auditing support with Spring Data JPA - Part 1 09:44
- 152 - Implement automatic auditing support with Spring Data JPA - Part 2 07:41
- 153 - Building new user registration web page inside Eazy School Web App 13:41
- 154 - Building Custom validations for new user registration page - Part 1 08:34
- 155 - Building Custom validations for new user registration page - Part 2 13:55
- 156 - Building Custom validations for new user registration page - Part 3 07:48
- 157 - Building Custom validations for new user registration page - Part 4 16:13
- 158 - Creating new tables required for new user registration process 10:37
- 159 - Spring Data JPA configurations for Person, Address, Roles tables and entities 08:13
- 160 - Introduction to One to One Relationship inside ORM frameworks 06:50
- 161 - Making One to One Relationship configurations inside entity classes - Theory 08:01
- 162 - Deep dive on Fetch Types and Cascade Types in ORM frameworks 10:04
- 163 - Making One to One Relationship configurations inside entity classes - Coding 17:23
- 164 - Understanding Spring Security configurations for custom authentication logic 09:03
- 165 - Implement Spring Security changes for custom authentication logic - Part 1 14:02
- 166 - Implement Spring Security changes for custom authentication logic - Part 2 07:05
- 167 - Problems with Authentication logic using plain text passwords 03:27
- 168 - Deep dive on Encoding, Encryption and Hashing for password management 12:00
- 169 - Deep dive on PasswordEncoder & BCryptPasswordEncoder 08:37
- 170 - Implementing password hashing with BCryptPaswordEncoder - Part 1 14:17
- 171 - Implementing password hashing with BCryptPaswordEncoder - Part 2 05:54
- 172 - Quick Tip - To Disable the javax validations in Spring Data JPA 05:54
- 173 - Displaying Profile link inside Dashboard web page 06:04
- 174 - Displaying Profile Web Page on click of profile link in Dashboard 12:29
- 175 - Fetch data from DB and display on the Profile web page 14:44
- 176 - Save Address Data into DB from Profile Page 09:24
- 177 - Introduction to new enhancements related to OnetoMany, ManytoOne & ManytoMany 02:19
- 178 - Displaying Classes, Courses link inside Dashboard web page 07:57
- 179 - Introduction to OneToMany & ManyToOne mappings 10:40
- 180 - Implement OneToMany & ManyToOne configurations inside Entity classes 09:07
- 181 - Displaying new Web Page on click of classes link in Dashboard 07:03
- 182 - Add & Delete Classes enhancement inside Eazy School Web App 13:22
- 183 - Display, Add & Delete Students enhancement inside Eazy School Web App - Part 1 14:17
- 184 - Display, Add & Delete Students enhancement inside Eazy School Web App - Part 2 08:48
- 185 - Introduction to ManyToMany relationship in ORM frameworks 12:54
- 186 - Implement ManyToMany configurations inside Entity classes 11:01
- 187 - Display & Add Courses enhancement inside Eazy School Web App - Part 1 08:36
- 188 - Display & Add Courses enhancement inside Eazy School Web App - Part 2 10:46
- 189 - Display & Add Students enhancement inside Course Web Page 11:21
- 190 - Delete Student enhancement inside Course Web Page 06:03
- 191 - Implement Student Dashboard related enhancements inside Eazy School Web App 14:43
- 192 - Introduction to Sorting inside Spring Data JPA 06:57
- 193 - Implement & Demo of Static Sorting 06:07
- 194 - Implement & Demo of Dynamic Sorting 05:12
- 195 - Introduction to Pagination inside Spring Data JPA 09:33
- 196 - Implement & Demo of Pagination & Dynamic Sorting - Part 1 21:03
- 197 - Implement & Demo of Pagination & Dynamic Sorting - Part 2 12:21
- 198 - Introduction to custom queries using @Query,@NamedQuery,@NamedNativeQuery & JPQL 13:56
- 199 - Writing Custom Queries using @Query Annotation 08:30
- 200 - Writing Custom Update Queries using @Query,@Modifying,@Transactional Annotations 12:11
- 201 - Deep dive on @NamedQuery,@NamedNativeQuery inside Spring Data JPA 05:46
- 202 - Writing Custom Queries using @NamedQuery,@NamedNativeQuery Annotations 15:17
- 203 - Introduction to REST Services 06:54
- 204 - Build REST services using Spring MVC style & @ResponseBody annotation - Theory 05:42
- 205 - Implement REST service using Spring MVC style & @ResponseBody - Part 1 08:41
- 206 - Implement REST service using Spring MVC style & @ResponseBody - Part 2 08:27
- 207 - Deep dive & Demo of @RequestBody annotation 10:03
- 208 - Implement REST Services using @RestController annotation 03:43
- 209 - Demo of save operation using Rest Service & ResponseEntity 13:47
- 210 - Demo of delete operation using Rest Service & RequestEntity 06:19
- 211 - Demo of update operation using Rest Service & recap of all Rest annotations 08:17
- 212 - Implement global error logic for Rest Services using @RestControllerAdvice 06:51
- 213 - Deep dive on CROSS-ORIGIN RESOURCE SHARING (CORS) & @CrossOrigin annotation 07:09
- 214 - Sending Response in XML format in Rest Services 05:03
- 215 - Demo of Content filter inside Rest Services using @JsonIgnore annotation 04:53
- 216 - Introduction to Consuming Rest Services inside Web Applications 09:55
- 217 - Consuming Rest Services using OpenFeign - Theory 10:01
- 218 - Consuming Rest Services using OpenFeign - Coding 11:37
- 219 - Consuming Rest Services using RestTemplate 14:33
- 220 - Consuming Rest Services using WebClient 15:13
- 221 - Introduction to Spring Data Rest & HAL Explorer 07:09
- 222 - Deep dive of Spring Data Rest & exploring Rest APIs - Part 1 10:49
- 223 - Deep dive of Spring Data Rest & exploring Rest APIs - Part 2 12:20
- 224 - Exploring Rest APIs of Spring Data Rest using HAL Explorer 08:58
- 225 - Securing Spring Data Rest APIs & HAL Explorer 03:43
- 226 - Quick Tips around Spring Data Rest 06:43
- 227 - Introduction to Logging inside SpringBoot 09:56
- 228 - Logging configurations for SpringBoot framework code 07:35
- 229 - Logging configurations for Application code 09:04
- 230 - Store log statements into a custom file and folder 12:01
- 231 - Introduction to Externalized properties inside SpringBoot Web Applications 06:39
- 232 - Reading properties using @Value annotation 07:18
- 233 - Reading properties using Environment interface 04:52
- 234 - Reading properties using @ConfigurationProperties - Theory 10:40
- 235 - Reading properties using @ConfigurationProperties - Coding 10:34
- 236 - Introduction to Profiles in Spring 04:31
- 237 - Implementation & Demo of Profiles inside Eazy School Web App 08:34
- 238 - Various approaches to activate Profiles inside Spring 14:07
- 239 - Creating beans conditionally based on active profile 06:27
- 240 - Introduction to Spring Boot Actuator 03:56
- 241 - Implement and Secure Actuator inside Eazy School Web App 06:39
- 242 - Deepdive of Actuator endpoints 17:20
- 243 - Exploring Actuator data using Spring Boot Admin 15:13
- 244 - Introduction to Cloud Deployment, AWS EC2 & AWS Elastic Beanstalk 09:31
- 245 - Packaging Spring Boot application for AWS Deployment 07:04
- 246 - Deploying Spring Boot app into AWS Elastic Beanstalk 11:27
- 247 - Switching DB inside AWS Elastic Beanstalk 08:27
- 248 - Deleting AWS Beanstalk & DB resources 03:06
- 249 - Thank You & Congratulations 02:32
About the Instructor
udemy
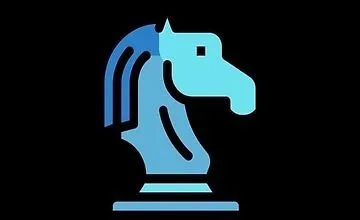
$0.00
$8.00 Que esta incluido?
- Streaming Multiplataforma
- Acceso de por vida
- Soporte al cliente
- Actualizaciones gratuitas