Python Jumpstart by Building 10 Apps
07:08:59 Inglés Free 01/05/2024 113 videos
Descripción del curso
Programming is fun and profitable. Learning to become a software developer should be equally fun! This course will teach you everything you need to know about the Python language all the while building interesting and engaging applications.
What's this course about and how is it different?
The goal of this online video course is to teach you the Python programming language.
It assumes you have just a small amount of programming experience (e.g. you know what a variable, a function, and a loop are in some language). But otherwise, it is a from the ground up, comprehensive introduction to the Python programming language.
Most courses focus on teaching you hundreds of details and leave putting them together as an exercise for the student. My course is different.
You will learn all the basics, yes. But you will learn them while building 10 stand alone applications. You will see each application built from the ground up in live demos. When we hit new topics (functions for example), we will pause, discuss them, and return to our application we are building.
This way you will continuously be "putting the pieces together". You don't have to wade through many small details before making sense of Python. It starts right from the beginning and grows from there.
Who is this course for?
It's for people who have some programming / scripting experience and want to improve their Python knowledge. Maybe you:
- Know JavaScript but you want to learn Python
- Used Python casually, but you want to learn it comprehensively
- Know part of the language well, but want rounded knowledge
- Want to write more Pythonic code (iterators, comprehensions, etc.)
- Are a scientist looking to use the Python data tools and need a foundation
- Are a college student and want more than your university course offers
- Considering becoming a software developer
- Hello world
- test your environment
- Guess that number
- user input
- conditionals
- string parsing
- Birthday app
- dates and times
- Personal journal
- text-based file i/o
- Weather client
- external packages
- pip
- screen scraping
- HTTP clients
- LOLCat Factory
- binary files on the internet
- Wizard battle
- classes
- inheritance
- magic methods
- File searcher app
- lambda expressions
- generator methods
- yield and yield from
- Real estate analyzer
- file formats
- list comprehensions
- generators expressions
- Movie lookup app
- error handling
- exceptions
- Advanced HTTP clients
Curriculum
Section 1: Module 1
About the Instructor
Talkpython
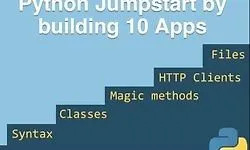
$0.00
$8.00 Que esta incluido?
- Streaming Multiplataforma
- Acceso de por vida
- Soporte al cliente
- Actualizaciones gratuitas