Test-Driven Laravel
21:48:56 Inglés Premium 02/04/2024 166 videos
Descripción del curso
The biggest objective of this course is to teach you how to TDD something real; not just another cookie-cutter to-do app.
We cover fundamentals like:
- What test should you write first
- Organizing your test suite
- Feature tests vs. unit tests
- Testing validation rules
- Testing events and background jobs
- Working with test databases
- Speeding up your tests with test doubles
- Testing code that interacts with third-party services
- Writing your own test doubles from scratch
- Testing automated payouts with Stripe Connect
- Testing the sending of mass emails
- How to test race conditions
- Testing file uploads and server side image processing
Curriculum
Section 1: Module 1
- 02 - What Do We Build First? 11:30
- 03 - Sketching out Our First Test 09:06
- 04 - Getting to Green 16:42
- 05 - Unit Testing Presentation Logic 11:03
- 06 - Refactoring for Speed 07:16
- 07 - Hiding Unpublished Concerts 06:56
- 08 - Testing Query Scopes 05:50
- 09 - Factory States 04:10
- 10 - Introducing the Next Feature 01:02
- 11 - Browser Testing vs Endpoint Testing 09:47
- 12 - Outlining the First Purchasing Test 06:23
- 13 - Faking the Payment Gateway 12:23
- 14 - Adding Tickets to Orders 05:32
- 15 - Encapsulating Relationship Logic in the Model 05:16
- 16 - Getting Started with Validation Testing 06:56
- 17 - Reducing Duplication with Custom Assertions 05:30
- 18 - Handling Failed Charges 07:00
- 19 - Preventing Ticket Sales to Unpublished Concerts 06:40
- 20 - Outlining the First Test Case 03:46
- 21 - Adding Tickets to Concerts 08:20
- 22 - Refusing Orders When There Are No More Tickets 09:29
- 23 - Finishing the Feature Test 04:25
- 24 - Cancelling Failed Orders 05:59
- 25 - Refactoring and Redundant Test Coverage 07:49
- 26 - Cleaning Up Our Tests 11:57
- 27 - Asserting Against JSON Responses 07:32
- 28 - Returning Order Details 06:22
- 29 - This Design Sucks 04:39
- 30 - Persisting the Order Amount 05:05
- 31 - Removing the Need to Cancel Orders 06:01
- 32 - Preparing for Extraction 07:06
- 33 - Extracting a Named Constructor 04:46
- 34 - Precomputing the Order Amount 05:23
- 35 - Uncovering a New Domain Object 05:11
- 36 - You Might Not Need a Mocking Framework 03:48
- 37 - Uh Oh, a Race Condition! 01:43
- 38 - Requestception 04:17
- 39 - Hooking into Charges 05:38
- 40 - Uh Oh, a Segfault! 03:01
- 41 - Replicating the Failure at the Unit Level 05:04
- 42 - Reserving Individual Tickets 07:00
- 43 - Reserved Means Reserved! 03:58
- 44 - That Guy Stole My Tickets! 05:59
- 45 - Cancelling Reservations 05:46
- 46 - Refactoring Mocks to Spies 06:47
- 47 - A Change in Behavior 05:53
- 48 - Deleting Stale Tests 05:38
- 49 - Cleaning up a Loose Variable 06:48
- 50 - Moving the Email to the Reservation 07:28
- 51 - Refactoring "Long Parameter List" Using "Preserve Whole Object" 09:50
- 52 - Green with Feature Envy 06:12
- 53 - Avoiding Service Classes with Method Injection 09:04
- 54 - Generating a Valid Payment Token 10:01
- 55 - Retrieving the Last Charge 04:05
- 56 - Making a Successful Charge 04:37
- 57 - Dealing with Lingering State 12:18
- 58 - Don't Mock What You Don't Own 09:41
- 59 - Using Groups to Skip Integration Tests 02:19
- 60 - Handling Invalid Payment Tokens 03:59
- 61 - The Moment of Truth 04:28
- 62 - When Interfaces Aren't Enough 04:29
- 63 - Refactoring Towards Duplication 12:38
- 64 - Capturing Charges with Callbacks 08:10
- 65 - Making the Tests Identical 07:42
- 66 - Extracting a Contract Test 07:42
- 67 - Extracting the Failure Case 08:24
- 68 - Upgrading to Laravel 5.4 04:49
- 69 - Removing the BrowserKit Dependency 10:26
- 70 - Sketching Out Order Confirmations 07:52
- 71 - Driving out the Endpoint 05:21
- 72 - Asserting Against View Data 05:46
- 73 - Extracting a Finder Method 06:51
- 74 - Making Static Data Real 08:49
- 75 - Deciding What to Test in a View 08:24
- 76 - Decoupling Data from Presentation 05:11
- 77 - Fixing the Test Suite 06:28
- 78 - Stubbing the Interface 06:28
- 79 - Updating Our Unit Tests 05:16
- 80 - Confirmation Number Characteristics 04:48
- 81 - Testing the Confirmation Number Format 07:29
- 82 - Ensuring Uniqueness 08:44
- 83 - Refactoring to a Facade 05:59
- 84 - Promoting Charges to Objects 17:49
- 85 - Leveraging Our Contract Tests 11:02
- 86 - Storing Charge Details with Orders 08:01
- 87 - Deleting More Stale Code 10:27
- 88 - Feature Test and JSON Updates 08:27
- 89 - Claiming Tickets When Creating Orders 09:12
- 90 - Assigning Codes When Claiming Tickets 10:54
- 91 - The Birthday Problem 11:55
- 92 - Integrating Hashids 11:44
- 93 - Dealing with Out of Sync Mocks 04:21
- 94 - Wiring It All Together 05:19
- 95 - Ready to Demo 02:52
- 96 - Using a Fake to Intercept Email 10:35
- 97 - Testing Mailable Contents 10:39
- 98 - Cleanup and Demo 02:40
- 99 - Testing the Login Endpoint 11:26
- 100 - Should You TDD Simple Templates? 04:42
- 101 - Namespacing Our Test Suite 06:31
- 102 - Getting Started with Laravel Dusk 05:22
- 103 - QA Testing the Login Flow 06:26
- 104 - Preventing Guests from Adding Concerts 06:24
- 105 - Adding a Valid Concert 14:20
- 106 - Validation and Redirects 09:49
- 107 - Converting Empty Strings to Null 04:21
- 108 - Reducing Noise with Form Factories 09:13
- 109 - Connecting Promoters and Concerts 06:49
- 110 - Autopublishing New Concerts 05:46
- 111 - Asserting Against View Objects 12:09
- 112 - Avoiding Sort-Sensitive Tests 05:47
- 113 - Refactoring Assertions with Macros 11:39
- 114 - Viewing the Update Form 05:29
- 115 - The First Update Test 09:27
- 116 - Driving Out Basic Concert Updates 09:14
- 117 - Restricting Updates to Unpublished Concerts 11:31
- 118 - Storing the Intended Ticket Quantity 06:26
- 119 - Updating the Other Tests 05:25
- 120 - Refactoring Away Some Test Duplication 07:52
- 121 - Creating Tickets at Time of Publish 08:28
- 122 - Custom Factory Classes 07:44
- 123 - Discovering a New Resource 07:35
- 124 - Creating Published Concerts 11:12
- 125 - Adding Concerts without Publishing 04:03
- 126 - Pushing Logic Out of the View 08:47
- 127 - More Custom Assertion Fun 06:20
- 128 - Calculating Tickets Sold 08:22
- 129 - Making the Progress Bar Work 08:58
- 130 - Total Revenue and a Relationship Bug 08:40
- 131 - Creating a Custom OrderFactory 11:28
- 132 - Asserting Against Sort Order 12:15
- 133 - Splitting Large Tests 03:41
- 134 - Storing Messages for Attendees 12:31
- 135 - Confirming That a Job Was Dispatched 09:27
- 136 - Unit Testing the Job 18:29
- 137 - Refactoring for Robustness 11:16
- 138 - Mailable Testing Refresher and Demo 04:11
- 139 - Upgrading to Laravel 5.5 07:41
- 140 - Faking Uploads and File Systems 10:43
- 141 - Storing Files and Comparing Content 09:16
- 142 - Validating Poster Images 07:45
- 143 - Optional Files and the Null Object Pattern 05:39
- 144 - Testing Events 10:41
- 145 - Testing the Event Listener 11:42
- 146 - Resizing the Posted Image 12:26
- 147 - Optimizing the Image Size 10:51
- 148 - Upgrading Laravel and Deleting Some Code 03:40
- 149 - Viewing an Unused Invitation 10:25
- 150 - Viewing Used or Invalid Invitations 07:29
- 151 - Registering with a Valid Invitation 12:46
- 152 - Registering with an Invalid Invitation 05:20
- 153 - Validating Promoter Registration 05:54
- 154 - Testing a Console Command 17:40
- 155 - Sending Promoters an Invitation Email 05:52
- 156 - Test-Driving the Email Contents 07:53
- 157 - Getting Cozy with Stripe Connect 10:55
- 158 - Authorizing with Stripe 10:05
- 159 - Exchanging Tokens 17:44
- 160 - Unit Testing Middleware 13:29
- 161 - Testing Callbacks with Invokables 09:43
- 162 - Testing That Middleware Is Applied 08:16
- 163 - Updating Factories and a Speed Trick 03:30
- 164 - Total Charges for a Specific Account 10:43
- 165 - Paying Promoters Directly 07:47
- 166 - Splitting Payments with Stripe 16:53
- 167 - It's Alive 04:36
About the Instructor
adamwathan
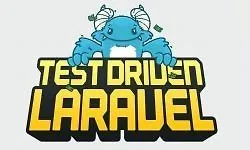
$0.00
$8.00 Que esta incluido?
- Streaming Multiplataforma
- Acceso de por vida
- Soporte al cliente
- Actualizaciones gratuitas