The Ultimate React Native Series: Advanced Concepts
05:28:42 Inglés Premium 06/06/2024 110 videos
Descripción del curso
Master advanced techniques and best practices for building professional-quality apps.
By the end of this course…
You’ll be able to:
- Build mobile apps with React Native with confidence
- Build beautiful user interfaces
- Build reusable components
- Write clean code like a pro
- Use essential tools for React Native development
- Properly structure your React Native projects
- Run and debug your React Native apps
- Understand and troubleshoot common errors
- Work with the core components and APIs
- Build layouts with Flexbox
- Build reusable components
- Apply beautiful styles to your components
- Get input from the user
- Build forms with Formik
- Implement data validation with Yup
- Publish your apps to Expo
- Apply React Native best practices
- Write clean code like a pro
- Use my favorite shortcuts to write code fast
- Access native device features
- Implement navigation using React Navigation
- Add beautiful tabs
- Communicate with REST APIs
- Upload images and show progress bars
- Build offline capable apps
- Cache data and images
- Implement authentication and authorization
- Send and receive push notifications
- Log and monitor errors
- Manage configuration settings across different environments
- Build and distribute your apps
Curriculum
Section 1: Module 1
About the Instructor
codewithmosh (Mosh Hamedani)
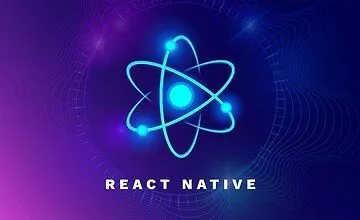
$0.00
$8.00 Que esta incluido?
- Streaming Multiplataforma
- Acceso de por vida
- Soporte al cliente
- Actualizaciones gratuitas